forked from interstellar_development/freettrpg
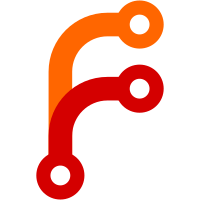
Second major release, minor tweaks, fully dynamic stats, now requires the official contentpack.
55 lines
1.5 KiB
GDScript
55 lines
1.5 KiB
GDScript
extends Node
|
|
|
|
var field = preload("res://scenes/menu/input-output.tscn")
|
|
var data:Dictionary
|
|
|
|
func loadJSON(save_path):
|
|
if not FileAccess.file_exists(save_path):
|
|
return false
|
|
var file_access = FileAccess.open(save_path, FileAccess.READ)
|
|
var json_string = file_access.get_line()
|
|
file_access.close()
|
|
|
|
var json = JSON.new()
|
|
var error = json.parse(json_string)
|
|
if error:
|
|
print("JSON Parse Error: ", json.get_error_message(), " in ", json_string, " at line ", json.get_error_line())
|
|
return false
|
|
|
|
data = json.data
|
|
return true
|
|
|
|
func _ready():
|
|
if loadJSON("res://content/stats.json"):
|
|
for i in range(int(data.get("amount"))):
|
|
var field_instance = field.instantiate()
|
|
add_child(field_instance)
|
|
field.resource_name = str("field_"+str(i))
|
|
for i in range(int(data.get("amount"))):
|
|
get_child(i+1).position = Vector2(16,(16+i*88))
|
|
get_child(i+1).placeholder_text = data.get(str(i))
|
|
|
|
func saveJSON(save_path):
|
|
var save_data = {}
|
|
|
|
for i in range(int(data.get("amount"))):
|
|
if i > 0:
|
|
save_data[data.get(str(i))] = get_child(i+1).text
|
|
|
|
var json_string = JSON.stringify(save_data)
|
|
|
|
var file_access = FileAccess.open(save_path, FileAccess.WRITE)
|
|
if not file_access:
|
|
print("An error happened while saving data: ", FileAccess.get_open_error())
|
|
return
|
|
|
|
file_access.store_line(json_string)
|
|
file_access.close()
|
|
|
|
func _on_button_pressed():
|
|
var save_path = str("user://player_data"+get_child(1).text+".json")
|
|
saveJSON(save_path)
|
|
|
|
func _process(delta):
|
|
if Input.is_action_pressed("escape"):
|
|
get_tree().change_scene_to_file("res://scenes/menu/main.tscn")
|